In the beginning, there were no programming languages. Things were done with the binary code which has 0 and 1 and looks exactly like this:
111 1110 0100 - This represents 2020, the year that Utopia Educators was created.
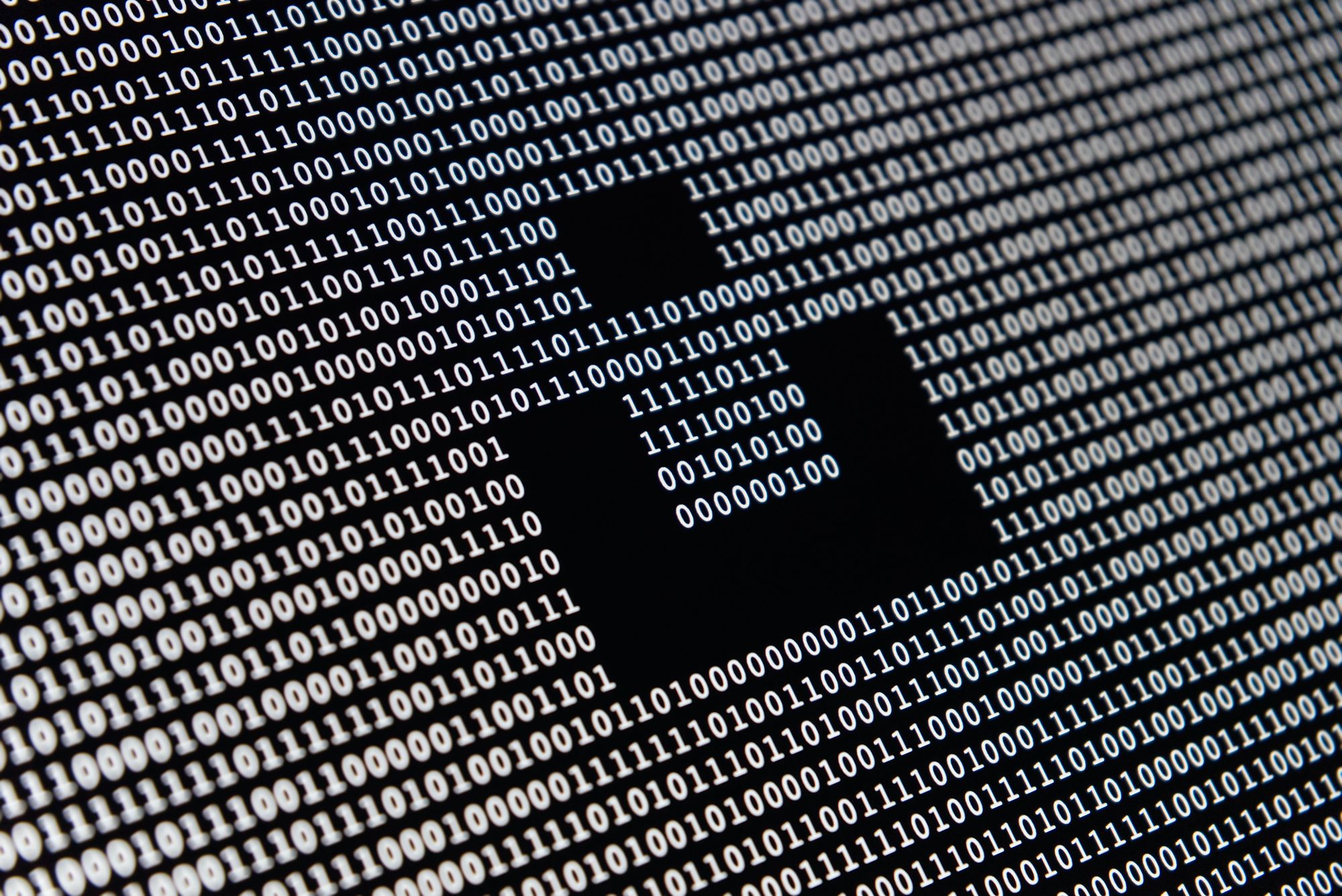
Nowadays we have programming languages that make our life a lot easier such as:
-C
-C++
-Python
-JavaScript
-PHP
-C#
Today, in this article we are going to give you a brief introduction to JavaScript.
JavaScript was founded in 1995 by Brendan Eich. The first JavaScript public release was integrated into the Netscape Navigator 2.0.JavaScript is a high-level language that came with dynamic typing, class functions, object orientation and it's the main core of the World Wide Web.
Values, Types, and Operators.
Inside the computer, there is only data which is represented by 0 and 1. You can read, create and modify data. They are a sequence of bits. For example, we can express the number 24 in bits using 0 and 1.
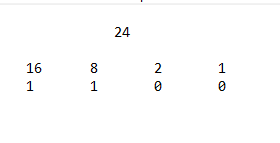
Since it's binary, the non-zero stand at 16 and 8 which adds up to 24.
Numbers
They are so common in daily use, JavaScript uses 64bits to store just a value. Which means there is a way to represent endless numbers. Computers memory used to be smaller so people used groups of 8 and 16 to represent a number.
Types of numbers:
-Fractional-uses dot. (Ex:4.5)
For big numbers, you might also find this: 1.921e8 is a scientific notation(for exponent), which is followed by the exponent.
Arithmetic
The best way to deal with numbers is arithmetic. There are arithmetic operators such as addition(+), multiplication(*), subtraction(-), division(/), but there is also a mod operator(%). By putting the operator between the numbers, it will show a new number. Example:

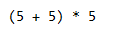
Strings
The next data type is a string. With it you can represent basic text. They are usually written in quotes. Example:
"Utopia Educator is the best website"
"You should subscribe for more interesting articles"
The escaping character(/)which is followed by n, is mostly used to go to a new line for example:
Input:
"Hello/nWorld"
Output:
"Hello"
"World"
Boolean Operators
It distinguishes possibilities like yes or no. They are also called true or false or 1 and o.Example:
console.log( 40 > 2)
"True"
console.log(40 < 2)
"False"
Logical operators:
- &&(AND operator)The answer is true if the 2 of them are true.
- ||(OR operator)either of the value is true or false.
- !(NOT operator)it flips the value that is given.
Functions
Defining a function
A function definition is a regular binding where the value of the binding is a function.
Example:

Nested scope
Blocks and functions can be created inside other blocks and functions, producing multiple degrees of the locality.
Functions as values
The function binding usually simply acts as a specific piece of the program. Such a binding is defined once and is never changed. This makes it easier to confuse the function and its name.
Closure
In closure, you can treat functions as types, combined with the fact that local bindings are re-created every time a function is called, which brings up an interesting question.
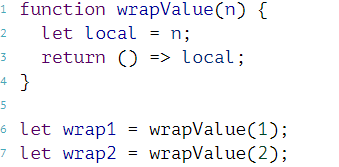
Recursion.
It allows some functions to have different style.